In this post, I will discuss how we can execute a SQL Query directly from LINQ.
Explanation
To execute SQL query there is a method in DataContext class called ExecuteQuery

ExecuteQuery takes two input parameter
1. SQL Query as string
2. Parameters used in SQL query

And it returns an IEnumerable.
Example
Below code will execute a simple select statement and it will return IEnumerable<Person>

If you want to pass some parameter in the query, you can pass that as second parameter.
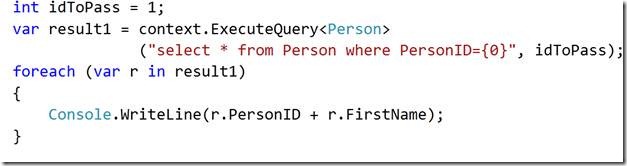
If you want pass input parameter as hardcoded value you can very much do that as below

For your reference source code is as below,
02 | using System.Collections.Generic; |
06 | using System.Data.Linq; |
08 | namespace Relatedtable |
13 | static DataClasses1DataContext context; |
14 | static void Main( string [] args) |
16 | context = new DataClasses1DataContext(); |
18 | var result = context.ExecuteQuery<Person>( "select * from Person" ); |
19 | foreach (var r in result) |
21 | Console.WriteLine(r.PersonID + r.FirstName); |
25 | var result1 = context.ExecuteQuery<Person> |
26 | ( "select * from Person where PersonID={0}" , idToPass); |
27 | foreach (var r in result1) |
29 | Console.WriteLine(r.PersonID + r.FirstName); |
32 | Console.ReadKey( true ); |
34 | var result2 = context.ExecuteQuery<Person> |
35 | ( "select * from Person where PersonID='1'" ); |
36 | foreach (var r in result2) |
38 | Console.WriteLine(r.PersonID + r.FirstName); |
41 | Console.ReadKey( true ); |
On pressing F5 you should get output as below,
![clip_image002[5] clip_image002[5]](http://dhananjay25.files.wordpress.com/2011/06/clip_image0025_thumb2.jpg?w=628&h=319)
I hope this post was useful. Thanks for reading 
0 Comments